How to Deploy Cloud Functions in Firebase
- Aarthi Krishnan
- May 26, 2024
- 4 min read
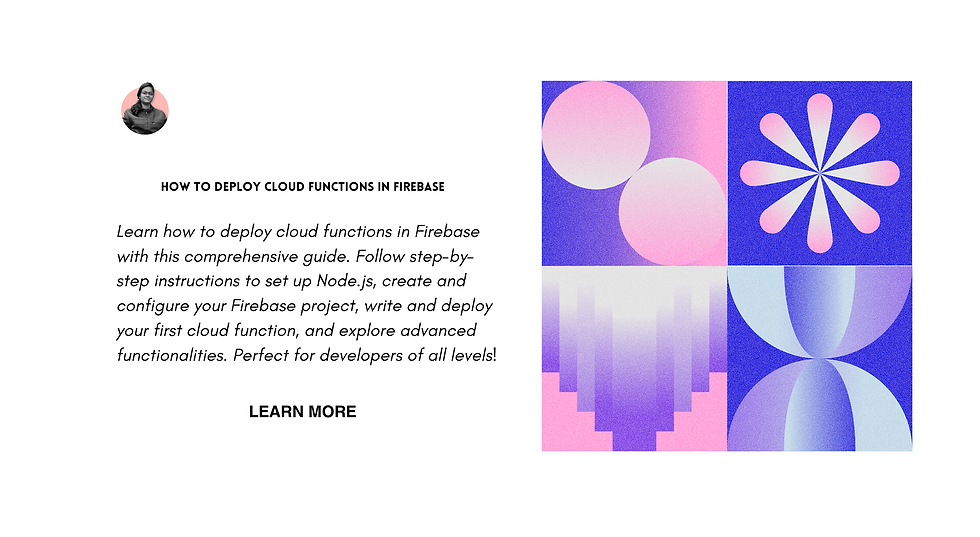
Introduction For Deploy Cloud Functions in Firebase
Welcome! I'm Aarthi Krishnan, and today I'll walk you through a detailed, step-by-step guide on deploying cloud functions in Firebase. By the end of this tutorial, you'll be well-equipped to deploy your own cloud functions, leveraging Firebase's robust platform. We'll cover everything from setting up the necessary tools to writing and deploying your first function. So, let's dive in!
Step 1: Setting Up Your Development Environment
Download and Install Node.js
To get started, you need to have Node.js installed on your system. Node.js is a JavaScript runtime that you'll use to write and deploy your Firebase functions. Download the latest version of Node.js from nodejs.org
Verification
After installing Node.js, open your terminal or command prompt and verify the installation by running the following commands:
node -v
npm -v
These commands will display the installed versions of Node.js and npm (Node Package Manager), confirming that the installation was successful.
Create a Firebase Account
If you haven't already, head over to Firebase and create an account. Firebase is a platform developed by Google for creating mobile and web applications. It provides a suite of tools and services to help you develop high-quality apps.
Upgrade to Blaze Plan
To deploy cloud functions, you need to upgrade to the Blaze plan (Pay as you go). Don't worry; you won't be charged unless you exceed the free tier usage. Follow these steps to upgrade:
1. Go to the Firebase Console.
2. Select your project or create a new one.
3. Navigate to the "Billing" section.
4. Choose the Blaze plan and enter your billing information.
Install Firebase CLI
The Firebase Command Line Interface (CLI) allows you to interact with Firebase services from the terminal. It's a powerful tool for deploying functions, managing projects, and more.
Installation
Install the Firebase CLI by running the following command in your terminal:
npm install -g firebase-tools
After installation, verify it by checking the version:
firebase --version
This command should output the version number of the installed Firebase CLI.
Step 2: Initialize Your Firebase Project
Set Up Your Project Directory
Next, create a directory for your Firebase project. This directory will contain all the files and configurations for your cloud functions.
Create Directory
Open your terminal and run:
mkdir firecast-deploy
cd firecast-deploy
This creates a new directory named `firecast-deploy` and navigates into it.
Log In to Firebase
Log in to your Firebase account using the CLI. This step authenticates your terminal session with Firebase.
Run the following command:
firebase login
This command will open a browser window where you need to log in with your Firebase credentials. Once logged in, return to the terminal.
Initialize Firebase in Your Project
Initialize your Firebase project within the `firecast-deploy` directory.
Initialization Command
firebase init
This command will start an interactive setup process. Follow these prompts:
1. Choose Firebase features: Select `Functions: Configure a Cloud Functions directory and its files`.
2. Select a project: Choose your Firebase project from the list.
3. Language choice: Select `JavaScript`.
4. ESLint: When prompted with "Do you want to use ESLint to catch probable bugs and enforce style?", select `No`.
5. Install dependencies: When asked "Do you want to install dependencies with npm now?", select `Yes`.
Upon completion, you'll see a message: "Firebase initialization complete!"
Step 3: Write Your First Cloud Function
Navigate to Functions Directory
Navigate to the `functions` directory created by Firebase initialization. This is where you'll write your cloud functions.
cd functions
Open `index.js` File
Open the `index.js` file in your preferred code editor. This file is the main entry point for your cloud functions.
Write the Function
Let's write a simple "Hello World" function.
Code Snippet
Replace the content of `index.js` with the following code:
const functions = require('firebase-functions');
exports.helloWorld = functions.https.onRequest((request, response) => {
response.send("Hello from Firebase!");
});
This code defines an HTTP function named `helloWorld`. When triggered, it sends back a "Hello from Firebase!" response.
Install Dependencies
Ensure all dependencies are installed. Run this command in the `functions` directory:
npm install
Step 4: Deploy Your Cloud Function
Deploy Command
Deploy your function to Firebase using the CLI. Run:
firebase deploy --only functions
This command will deploy only the cloud functions specified in your project.
Verify Deployment
After deployment, the CLI will output URLs for your deployed functions. You should see a URL for the `helloWorld` function. Visit this URL in your browser to test it. You should see the message "Hello from Firebase!".
Step 5: Explore the Firebase Console
Navigate to Functions Dashboard
In the Firebase Console, go to your project and navigate to the `Functions` section under `Build`. Here, you can manage and monitor your deployed functions.
Monitor Function Usage
Firebase provides detailed usage metrics for each function, including invocation counts, execution times, and error rates. Use this data to optimize and debug your functions.
Step 6: Advanced Functionality
Adding More Functions
You can add more functions to the `index.js` file. For example, add a new function that listens to Firebase Realtime Database events:
exports.onDataWrite = functions.database.ref('/path/{id}')
.onWrite((change, context) => {
const beforeData = change.before.val(); // data before the write
const afterData = change.after.val(); // data after the write
// Perform desired operations
return null;
});
This function triggers whenever data at `/path/{id}` is written to.
Scheduling Functions
Firebase also supports scheduled functions. Add a scheduled function to `index.js`:
exports.scheduledFunction = functions.pubsub.schedule('every 5 minutes').onRun((context) => {
console.log('This will run every 5 minutes!');
return null;
});
This function runs every 5 minutes as per the specified schedule.
Conclusion
You've now learned how to set up, write, and deploy cloud functions using Firebase. This powerful feature allows you to run backend code in response to events triggered by Firebase features and HTTPS requests. Whether you're building simple microservices or complex backend logic, Firebase Cloud Functions offer a scalable and efficient solution.
Here’s a recap of what we covered In this Deploy Cloud Functions in Firebase :
1. Setting up Node.js and Firebase.
2. Initializing a Firebase project.
3. Writing and deploying your first cloud function.
4. Exploring the Firebase Console for function management.
5. Advanced functionality with additional functions and scheduling.
By following these steps, you can take full advantage of Firebase Cloud Functions to enhance your application's capabilities. I hope this tutorial was helpful. Thank you for following along, and happy coding!

- Aarthi Krishnan
댓글